- Gcc Compiler Download For Mac
- Install Gcc Python For Mac Os
- Install Gcc Python Mac
- Install Gcc Python For Machine Learning
Pip install pygccxml. To install from source, you can use the usual procedure: python setup.py install. GCC-XML (Legacy)¶. These instructions are only here for historical reasons. GCC-XMLwas the tool usedto generate the xml files before CastXML existed. From version v1.8.0 on, pygccxml uses CastXML by default. You need GCC and a Python environment installed in order to build and install XGBoost for Python. I recommend GCC 7 and Python 3.6 and I recommend installing these prerequisites using MacPorts. For help installing MacPorts and a Python environment step-by-step, see this tutorial. Installing statsmodels¶ The easiest way to install statsmodels is to install it as part of the Anaconda distribution, a cross-platform distribution for data analysis and scientific computing. This is the recommended installation method for most users. Instructions for installing from PyPI, source or a development version are also provided.
The plugin has the following requirements:
GCC: 4.6 or later (it uses APIs that weren’t exposed to plugins in 4.5)
Python: requires 2.7 or 3.2 or later
“six”: The libcpychecker code uses the “six” Python compatibility library tosmooth over Python 2 vs Python 3 differences, both at build-time andrun-time:
“pygments”: The libcpychecker code uses the “pygments” Pythonsyntax-highlighting library when writing out error reports:
“lxml”: The libcpychecker code uses the “lxml” internally when writingout error reports.
graphviz: many of the interesting examples use “dot” to draw diagrams(e.g. control-flow graphs), so it’s worth having graphviz installed.
Various distributions ship with pre-built copies of the plugin. If you’reusing Fedora, you can install the plugin via RPM on Fedora 16 onwards using:
as root for the Python 2 build of the plugin, or:
for the Python 3 build of the plugin.
On Gentoo, use layman to add the dMaggot overlay and emerge thegcc-python-plugin package. This will build the plugin for Python 2 andPython 3 should you have both of them installed in your system. A liveebuild is also provided to install the plugin from git sources.
Build-time dependencies¶
If you plan to build the plugin from scratch, you’ll need the build-timedependencies.
On a Fedora box you can install them by running the following as root:
for building against Python 2, or:
when building for Python 3.
Building the code¶
You can obtain the source code from git by using:
To build the plugin, run:
To build the plugin and run the selftests, run:
You can also use:
to demonstrate the new compiler errors.
By default, the Makefile builds the plugin using the first python-config
tool found in $PATH (e.g. /usr/bin/python-config), which is typically thesystem copy of Python 2. You can override this (e.g. to build againstPython 3) by overriding the PYTHON and PYTHON_CONFIG Makefile variableswith:
There isn’t a well-defined process yet for installing the plugin (though therpm specfile in the source tree contains some work-in-progress towards this).
Some notes on GCC plugins can be seen at http://gcc.gnu.org/wiki/plugins andhttp://gcc.gnu.org/onlinedocs/gccint/Plugins.html
Note
Unfortunately, the layout of the header files for GCC plugindevelopment has changed somewhat between different GCC releases. Inparticular, older builds of GCC flattened the “c-family” directory in theinstalled plugin headers.
This was fixed in this GCC commit:
So if you’re using an earlier build of GCC using the old layout you’ll needto apply the following patch (reversed with “-R”) to the plugin’s sourcetree to get it to compile:
If you have a way to make the plugin’s source work with either layout,please email the plugin’s mailing list
Once you’ve built the plugin, you can invoke a Python script like this:
and have it run your script as the plugin starts up.
Alternatively, you can run a one-shot Python command like this:
such as:
The plugin automatically adds the absolute path to its own directory to theend of its sys.path, so that it can find support modules, such as gccutils.pyand libcpychecker.
There is also a helper script, gcc-with-python, which expects a python scriptas its first argument, then regular gcc arguments:
For example, this command will use graphviz to draw how GCC “sees” theinternals of each function in test.c (within its SSA representation):
Most of the rest of this document describes the Python API visible forscripting.
The plugin GCC’s various types as Python objects, within a “gcc” module. Youcan see the API by running the following within a script:
To make this easier, there’s a script to do this for you:
from where you can review the built-in documentation strings (this documentmay be easier to follow though).
The exact API is still in flux: and may well change (this is an early versionof the code; we may have to change things as GCC changes in future releasesalso).
Debugging your script¶
You can place a forced breakpoint in your script using this standard Pythonone-liner:
If Python reaches this location it will interrupt the compile and put youwithin the pdb interactive debugger, from where you can investigate.
See http://docs.python.org/library/pdb.html#debugger-commands for moreinformation.
If an exception occurs during Python code, and isn’t handled by a try/exceptbefore returning into the plugin, the plugin prints the traceback to stderr andtreats it as an error:
(In this case, it was a missing import statement in the script)
GCC reports errors at a particular location within the source code. For anunhandled exception such as the one above, by default, the plugin reportsthe error as occurring as the top of the current source function (or the lastlocation within the current source file for passes and callbacks that aren’tassociated with a function).
You can override this using gcc.set_location:

gcc.
set_location
(loc)¶Temporarily overrides the error-reporting location, so that if an exceptionoccurs, it will use this gcc.Location, rather than the default. This maybe of use when debugging tracebacks from scripts. The location is reseteach time after returning from Python back to the plugin, after printingany traceback.
Accessing parameters¶
gcc.
argument_dict
¶Exposes the arguments passed to the plugin as a dictionary.
For example, running:
with test.py containing:
has output:
gcc.
argument_tuple
¶Exposes the arguments passed to the plugin as a tuple of (key, value) pairs,so you have ordering. (Probably worth removing, and replacingargument_dict
with an OrderedDict instead; what aboutduplicate args though?)
Adding new passes to the compiler¶
You can create new compiler passes by subclassing the appropriategcc.Pass
subclasss. For example, here’s how to wire up a new passthat displays the control flow graph of each function:
For more information, see Creating new optimization passes
Wiring up callbacks¶
The other way to write scripts is to register callback functionsto be called when various events happen during compilation, such as usinggcc.PLUGIN_PASS_EXECUTION
to piggyback off of an existing GCC pass.
For more information, see Working with callbacks
gcc.
get_variables
()¶Get all variables in this compilation unit as a list ofgcc.Variable
gcc.
Variable
¶Wrapper around GCC’s struct varpool_node, representing a variable inthe code being compiled.
decl
¶The declaration of this variable, as a gcc.Tree
gccutils.
get_variables_as_dict
()¶Get a dictionary of all variables, where the keys are the variable names(as strings), and the values are instances of gcc.Variable
gcc.
maybe_get_identifier
(str)¶Get the gcc.IdentifierNode
with this name, if it exists,otherwise None. (However, after the front-end has run, the identifiernode may no longer point at anything useful to you; seegccutils.get_global_typedef()
for an example of workingaround this)
gcc.
get_translation_units
()¶Get a list of all gcc.TranslationUnitDecl
for the compilationunits within this invocation of GCC (that’s “source code files” for thelayperson).
TranslationUnitDecl
¶Subclass of gcc.Tree
representing a compilation unit
block
¶The gcc.Block
representing global scope within thissource file.
language
¶The source language of this translation unit, as a string(e.g. “GNU C”)
gcc.
get_global_namespace
()¶C++ only: locate the gcc.NamespaceDecl
for the globalnamespace (a.k.a. “::”)
gccutils.
get_global_typedef
(name)¶Given a string name, look for a C/C++ typedef in global scope withthat name, returning it as a gcc.TypeDecl
, or None if itwasn’t found
Gcc Compiler Download For Mac
gccutils.
get_global_vardecl_by_name
(name)¶Install Gcc Python For Mac Os
Given a string name, look for a C/C++ variable in global scope withthat name, returning it as a gcc.VarDecl
, or None if itwasn’t found
gccutils.
get_field_by_name
(decl, name)¶Install Gcc Python Mac
Given one of a gcc.RecordType
, gcc.UnionType
, orgcc.QualUnionType
, along with a string name, look for afield with that name within the given struct or union, returning it as agcc.FieldDecl
, or None if it wasn’t found
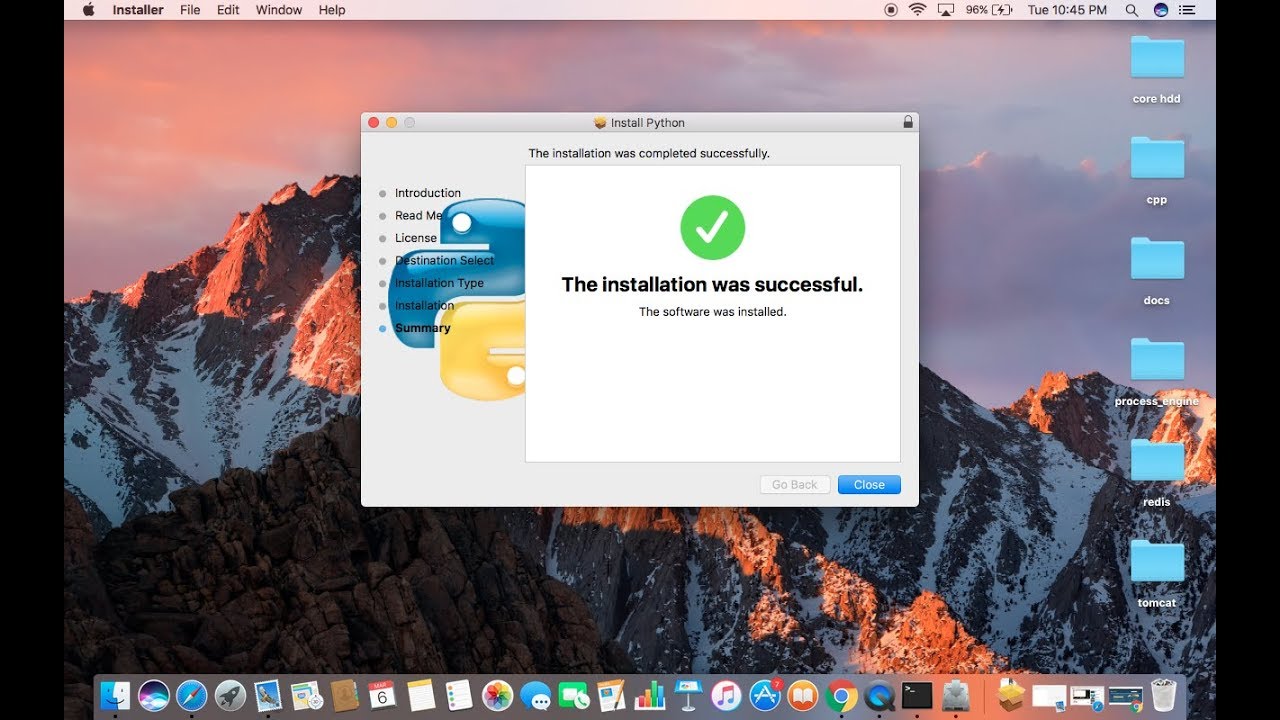
Dependencies¶
The Javabridge requires Python 2.6 or above, NumPy, the JavaDevelopment Kit (JDK), and a C compiler.
Linux¶
On CentOS 6, the dependencies can be installed as follows:
On Fedora 19, the dependencies can be installed as follows:
Install Gcc Python For Machine Learning
On Ubuntu 13 and Debian 7, the dependencies can be installed as follows:
On Ubuntu 14, the dependencies can be installed as follows:
On Arch Linux, the dependencies can be installed as follows:
MacOS X¶
- Install the Xcode command-line tools. There are two ways:
- Install Xcode from the Mac App Store. (You can also download itfrom Apple’s Mac Dev Center, but that may require membership inthe Apple Developer Program.) Install the Xcode command-linetools by starting Xcode, going to Preferences, click on“Downloads” in the toolbar, and click the “Install” button onthe line “Command Line Tools.” For MacOS 10.9 and Xcode 5 andabove, you may have to install the command-line tools by typing
xcode-select--install
and following the prompts. - Download the Xcode command-line tools from Apple’s Mac DevCenter and install. This may require membership in the AppleDeveloper Program.
- Install Xcode from the Mac App Store. (You can also download itfrom Apple’s Mac Dev Center, but that may require membership inthe Apple Developer Program.) Install the Xcode command-linetools by starting Xcode, going to Preferences, click on“Downloads” in the toolbar, and click the “Install” button onthe line “Command Line Tools.” For MacOS 10.9 and Xcode 5 andabove, you may have to install the command-line tools by typing
- Create and activate a virtualenv virtual environment if you don’twant to clutter up your system-wide python installation with newpackages.
pipinstallnumpy
pipinstalljavabridge
Windows¶
If you do not have a C compiler installed, you can install the WindowsSDK 7.1 and .Net Framework 4.0 to perform the compile steps.
You should install a Java Development Kit (JDK) appropriate for yourJava project. The Windows build is tested with the Oracle JDK 1.7. Youalso need to install the Java Runtime Environment (JRE). Note thatthe bitness needs to match your python: if you use a 32-bit Python,then you need a 32-bit JDK; if you use a 64-bit Python, then you needa 64-bit JDK.
The paths to PIP and Python should be in your PATH (setPATH=%PATH%;c:Python27;c:Python27scripts
if Python and PIPinstalled to the default locations). The following steps shouldperform the install:
Open a Windows SDK command prompt (found in the Start menu underMicrosoft Windows SDK). Set the path to Python and PIP if needed.
Issue the commands:
